Table of Contents
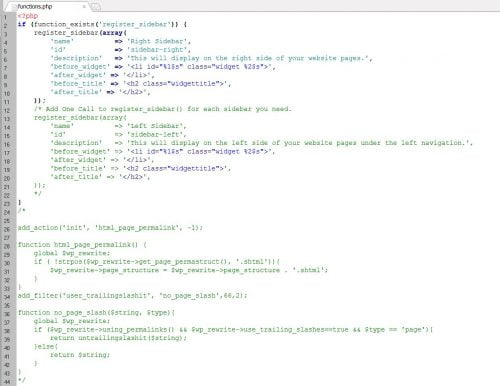
Here are some useful PHP array functions.
Here is the list of PHP array functions. If you are a beginner and wanted to know the basic PHP functions then navigate your browser to the “Most Useful PHP Functions with Examples” post.
sizeof () This function is used to count the items of the array. (count() is also used instead of this).
Example
<?php
//Define array
$data=array(
“Bugatti”,
“Gallardo”,
“Audi”,
“Nissan”,
” Chevrolet “);
Â
echo sizeof($data);
?>
Â
explode () This function is used to explode the string into an array.
Example
<?php
//Define string
$str=”apple,microsoft,android,ubuntu”
$fruits=explode(“,”,$str);
print_r($fruits);Â Â Â Â Â Â Â Â Â Â Â Â Â Â Â //print_r() is used to get the data type
?>
Â
implode () This function is used to convert the array into a string.
Example
<?php
//Define array
$data=array(
“Dell”,
“Lenovo”,
“Acer”,
“Toshiba”);
Â
//Convert array into string by using any delimeter
$str=implode(” and “, $data);
print_r($str)
?>
Â
range () This function allows us to get the range of numbers such as 1,10 or 1,100 etc.. We need to specify two points. First one is the starting point and the second one is the endpoint. It returns all the numbers from the specified range.
Example
<?php
//Define number range
$ran=range(1,10);
foreach($ran as $r){
echo $r;
}
?>
Â
min () max () These functions are used to get the smallest and largest numbers in specified array.
Example
<?php
//Define array
$data=array(1,10,4,6,7,9,2);
echo “Smallest number in this array is ” . min($data) . ” and largest number in this array is ” . max($data) . “.”;
?>
Â
array_slice () This function is used to extract the particular segment from an array. It accepts 3 arguments. The original array, the starting index of array and the number of arrays to return. (If you want to slice the array from the end then use negative (-) symbol and the main thing is that we don’t use 0,1,2 index when counting from end but we use 1,2 index).
Example
<?php
//Define array
$data=array(1,10,4,6,7,9,2);
$arr=array_slice($data,1,3);
print_r($arr);Â Â Â Â //This is returned as an array
?>
Â
array_unshift () This function is used to add the element at the beginning of the array.
Â
array_shift () This function is used to remove the element from the beginning of the array.
Â
array_push () This function is used to add the element at the end of the array.
Â
array_pop () This function is used to remove the element from the end of the array.
Â
array_unique () This function is used to remove the duplicate elements from the array. The below example makes a new array called $un without having the duplicate arrays from the array $data.
Example
<?php
$data=array(“a”,”x”,”s”,”z”,”a”,”z”);
$un=array_unique($data);
print_r($un);
?>
Â
shuffle () This function is used to shuffle (randomize) the array. (No need to make a new array data type).
Â
array_reverse () This function is used to reverse the array. (Need to make a new array data type).
Â
in_array () This function is used to search element in an array. It returns true if the specified element is exist in the array. It accepts 2 arguments – the element to search and the array data type. (If we want to search the keys then we have to use array_key_exists ()
Example
<?php
$data=array(“a”,”x”,”s”,”z”,”a”,”z”);
echo in_array(“a”,$data);
?>
Â
sort () This function is used to sort the array. (If we want to reverse the sort sequence then we have to use rsort ())
Example
<?php
$data=array(3,5,1,8,2,4,6,7);
sort($data);
foreach($data as $d){
echo $d . “\r\n”;
}
?>
Â
asort () This function is used to sort the associative array. Values are sorted here. (If we want to reverse the sort sequence then we have to use arsort ())
Â
ksort () This function is used to sort the associative array. keys are sorted here. (If we want to reverse the sort sequence then we have to use krsort ())
Â
array_intersect () This function is used to find the common elements in two arrays. It accepts two argments (1st array and 2nd array).
array_diff () This function returns the values of the first array which are not exist in second. It accepts two argments (1st array and 2nd array).
Example
<?php
$fstarray=array(“Red”, “Green”, “Blue”);
$secarray=array(“Pink”,”Green”,”Yellow”, “Red”);
$common= array_intersect($fstarray,$secarray);
foreach($common as $c){
echo $c . “\r\n”;
}
echo “<br />”;
$unique=array_diff($fstarray,$secarray);
foreach($unique as $u){
echo $u . “\r\n”;
}
?>